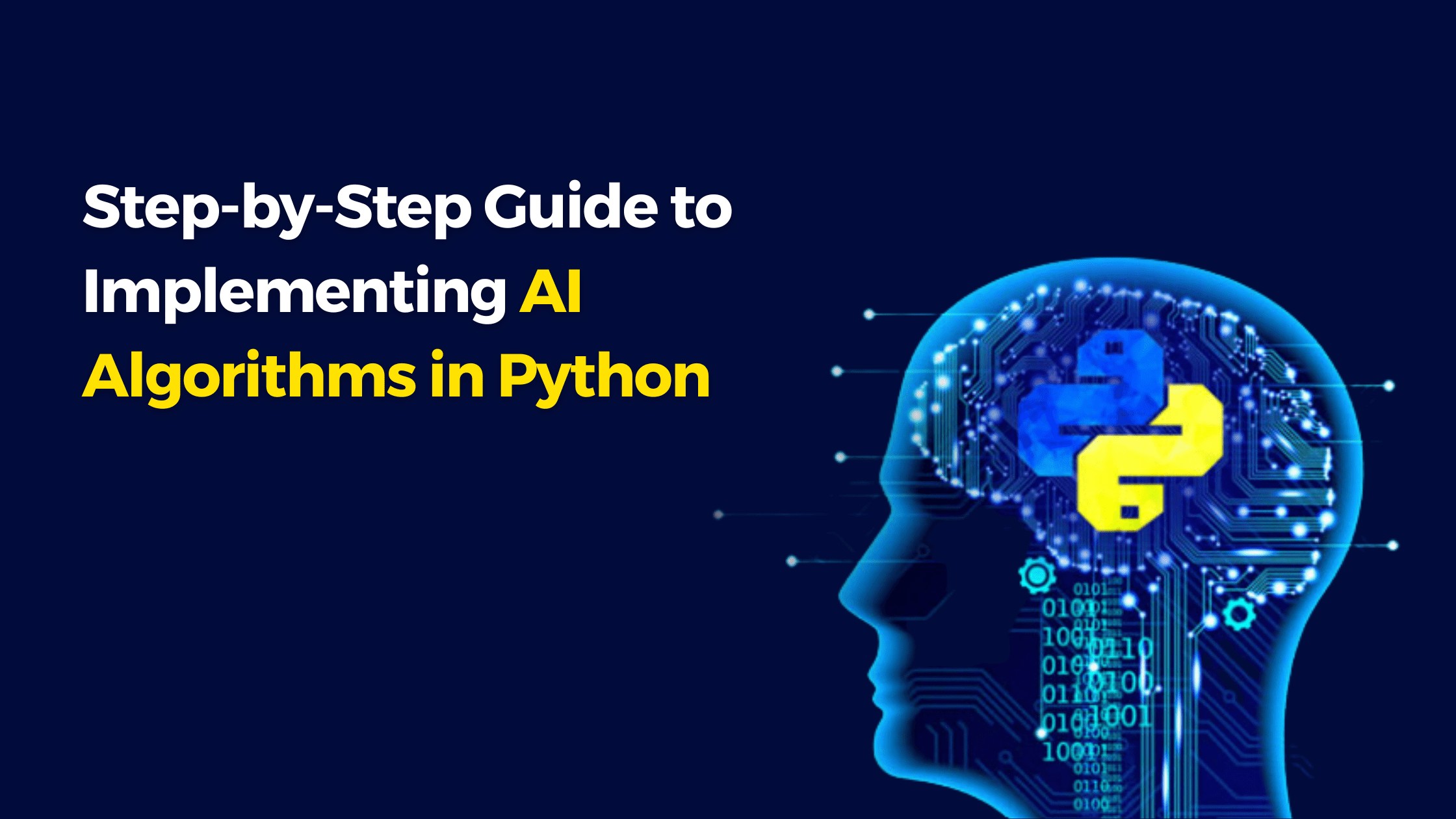
Welcome to the exciting world of artificial intelligence! Whether you’re a seasoned programmer or just dipping your toes into coding, implementing AI algorithms in Python is an incredible journey that can open up endless possibilities. From machine learning to natural language processing, this step-by-step guide will take you by the hand and show you how to bring these powerful algorithms to life. Get ready to unleash the potential of AI as we explore the realm where code meets intelligence, one line at a time. Let’s dive in and transform your programming prowess into cutting-edge AI innovation!
Introduction to Artificial Intelligence and Python
Artificial Intelligence (AI) is a rapidly growing field that focuses on creating intelligent machines that can perform tasks that typically require human intelligence. These tasks include learning, problem-solving, perception, reasoning, and decision making. With the advancements in technology and the increasing availability of data, AI has become an essential component in various industries such as healthcare, finance, marketing, and transportation.
Python is a popular programming language that has gained widespread usage in the field of AI due to its simplicity and flexibility. It offers a vast array of libraries and tools specifically designed for implementing AI algorithms efficiently. In this section, we will provide an overview of both artificial intelligence and Python to help you understand how they work together.
Understanding Artificial Intelligence
Artificial Intelligence can be broadly categorized into two types – Narrow AI (also known as weak AI) and General AI (also known as strong AI). Narrow AI refers to systems designed for specific tasks or narrow domains such as image recognition or natural language processing. On the other hand, General AI aims to create machines with human-like intelligence capable of performing any cognitive task.
To enable computers to simulate human intelligence effectively, it requires three fundamental components: learning from data through algorithms, reasoning based on knowledge representation models like logic or probability theory, and self-correction through feedback loops. These components are combined using advanced techniques such as Machine Learning (ML), Natural Language Processing (NLP), Computer Vision (CV), Robotics Process Automation (RPA), etc.,
Benefits of Using Python for AI
There are numerous programming languages that can be used for Artificial Intelligence (AI) development, but Python has emerged as one of the most popular and widely used languages in this field. Its simplicity, versatility, and powerful libraries make it an ideal choice for implementing AI algorithms. In this section, we will discuss some of the key benefits of using Python for AI.
1. Easy to Learn and Use:
Python is a high-level programming language that was designed with readability in mind. This means that its syntax is simple and easy to understand, making it accessible even to those who have little or no background in coding. Additionally, Python’s extensive documentation and large community make it easier for beginners to learn and get support when needed.
2. Versatility:
One of the main advantages of Python is its versatility – it can be used for a wide range of applications including web development, data analysis, machine learning, and AI. This makes it a valuable skill to have as a programmer since you can apply your knowledge to various projects without having to learn new languages.
3. Rich Library Ecosystem:
Python has an extensive collection of libraries dedicated specifically to AI development such as TensorFlow, Keras, PyTorch, Scikit-learn among others. These libraries provide ready-made modules for tasks like data manipulation, visualization, and model building which significantly speeds up the development process.
4. Scalability:
Python’s scalability allows developers to easily handle complex tasks involving large datasets without compromising on performance or speed – a crucial factor
Basic Concepts of AI Algorithms
Before diving into the implementation of AI algorithms in Python, it is important to have a clear understanding of the basic concepts behind them. This section will cover the fundamental principles and terminology used in artificial intelligence and machine learning, which are essential for successfully implementing AI algorithms.
1. Artificial Intelligence (AI):
AI refers to the development of computer systems that can perform tasks that typically require human intelligence. It encompasses a wide range of techniques and approaches including machine learning, natural language processing, computer vision, and robotics.
2. Machine Learning (ML):
Machine learning is a subset of AI that focuses on enabling machines to learn from data without being explicitly programmed. It involves creating algorithms that can automatically improve through experience by identifying patterns or trends in data.
3. Supervised Learning:
Supervised learning is a type of ML algorithm where the model learns from labeled data to make predictions or decisions about new data. The goal is to find patterns in the input-output relationships presented in the training dataset so that it can accurately predict outputs for unseen inputs.
4. Unsupervised Learning:
Unsupervised learning is another type of ML algorithm where the model learns from unlabeled data without any predefined output labels. The goal is to find hidden structures or patterns within the data itself without any prior knowledge or guidance.
5. Reinforcement Learning:
Reinforcement learning involves training an agent through trial-and-error interactions with an environment to maximize rewards for certain actions taken by the agent. This approach has been successfully applied in various fields such as
– Machine Learning
Machine learning is a subset of artificial intelligence that focuses on developing algorithms and techniques that enable computers to learn from data, without explicitly being programmed. This means that instead of providing specific instructions to the computer, we can feed it large amounts of data and let it “learn” patterns and make predictions or decisions based on that data.
There are three main types of machine learning: supervised learning, unsupervised learning, and reinforcement learning. In this section, we will discuss each type in detail and how you can implement them in Python.
1. Supervised Learning:
Supervised learning involves training a model with labeled data, where the input variables (features) and output variable (target) are already known. The goal is for the model to learn a general mapping between the input and output variables so that it can accurately predict the output for new inputs. Some common algorithms used in supervised learning include linear regression, decision trees, random forests, and neural networks.
To implement supervised learning algorithms in Python, you will need to use libraries such as scikit-learn or TensorFlow. These libraries provide pre-implemented functions for various supervised learning tasks like classification or regression. You will also need to have a good understanding of concepts like feature engineering (selecting relevant features), model evaluation techniques (e.g., cross-validation), and hyperparameter tuning (choosing optimal values for parameters).
2. Unsupervised Learning:
In unsupervised learning, there is no target variable provided; instead, the algorithm tries to find
– Deep Learning
Deep learning is a subset of artificial intelligence that focuses on teaching computers to learn and make decisions based on large amounts of data. It is inspired by the structure and function of the human brain, specifically its neural networks, which are complex interconnected systems that process information.
In recent years, deep learning has gained popularity due to advancements in computer processing power and availability of big data. With these resources, deep learning algorithms can be trained to recognize patterns and make predictions with high accuracy. In fact, some deep learning models have surpassed human performance in tasks like image recognition and natural language processing.
Nowadays, there are various frameworks available for implementing deep learning algorithms in Python. Some popular ones include TensorFlow, PyTorch, Keras, and Theano. These frameworks provide pre-built functions for building neural networks and training them on specific datasets.
One important aspect of deep learning is understanding the different types of neural networks used in this field. Convolutional Neural Networks (CNNs) are commonly used for image recognition tasks while Recurrent Neural Networks (RNNs) excel in sequential data analysis such as speech recognition or natural language processing.
To implement a deep learning algorithm in Python using any framework, the following are the general steps involved:
1. Data Preparation: The first step is to collect or generate a dataset suitable for training your model. This could involve cleaning, preprocessing, and organizing the data to be fed into the network.
2. Building the Model: Once you have your dataset ready, it’s time
– Natural Language Processing
Natural Language Processing (NLP) is a branch of artificial intelligence that involves teaching computers to understand, interpret and generate human language. It combines techniques from various fields such as computer science, linguistics, and statistics to enable machines to process, analyze and derive meaning from natural language data.
In recent years, NLP has gained significant traction due to the increasing amount of text data available on the internet. From social media posts and customer reviews to news articles and email communication, there is no shortage of textual information for machines to learn from. With the help of NLP algorithms, we can now automate tasks such as sentiment analysis, language translation, text summarization, and even chatbots.
To implement NLP algorithms in Python, we will need a few key libraries – NLTK (Natural Language Toolkit), spaCy, and Gensim. These libraries provide us with powerful tools for text processing and analysis. Let’s take a closer look at each of them:
1. NLTK: NLTK is one of the most popular open-source platforms for natural language processing in Python. It provides an easy-to-use interface for tasks like tokenization (breaking down a sentence into words or phrases), stemming (reducing words to their root form), part-of-speech tagging (labeling words by their grammatical category) and named entity recognition (identifying proper nouns). In addition to these basic functionalities, NLTK also offers numerous datasets for training models on specific tasks like sentiment analysis or language identification.
Step-by-Step Guide to Implementing AI Algorithms in Python:
Python has become one of the most popular programming languages for artificial intelligence (AI) and machine learning (ML) applications. Its simplicity, versatility, and wide range of libraries have made it a go-to choice for developers looking to implement AI algorithms. In this guide, we will walk you through the step-by-step process of implementing AI algorithms in Python.
1. Define the Problem:
The first step in implementing any AI algorithm is to clearly define the problem you want to solve. This involves understanding the data you have, identifying the desired outcome, and defining any constraints or limitations. Once you have a clear understanding of the problem at hand, you can move on to selecting an appropriate algorithm.
2. Choose an Appropriate Algorithm:
There are various types of AI algorithms such as supervised learning, unsupervised learning, and reinforcement learning. Each type is suitable for different types of problems. For example, if you have a dataset with labeled data (input-output pairs), then supervised learning algorithms like linear regression or decision trees would be more suitable. On the other hand, if your dataset does not have labels and you want to uncover patterns within it, then unsupervised learning algorithms like k-means clustering or principal component analysis (PCA) would be more appropriate.
3. Prepare Your Data:
Data preparation is crucial in building successful AI models as it directly impacts their performance.
– Setting up the Environment
Setting up the environment is a crucial step in implementing AI algorithms in Python. It involves installing the necessary software and configuring your system to run AI programs smoothly. In this section, we will go through the steps of setting up your environment for AI development.
1. Choose an IDE (Integrated Development Environment):
An IDE is a software application that provides a comprehensive set of tools for writing, testing, and debugging code. There are several options available for Python development such as PyCharm, Visual Studio Code, and Jupyter Notebook. Choose one that suits your needs and preferences.
2. Install Python:
Python is the primary language used for AI development due to its simplicity and vast community support. You can download and install the latest version of Python from their official website or by using package managers like Anaconda or Miniconda.
3. Set up a virtual environment:
Virtual environments allow you to create isolated environments with their own set of dependencies for different projects. This ensures that changes made in one project do not affect others. To set up a virtual environment, you can use python’s built-in venv module or third-party tools like Virtualenv or Conda.
4. Installing necessary libraries:
For AI development, you will need various libraries such as NumPy, Pandas, Scikit-learn, TensorFlow, Keras, etc., depending on your project requirements. These libraries provide powerful functions and methods to handle data manipulation, visualization, machine learning models, and more.
– Importing Necessary Libraries
Importing necessary libraries is a crucial step in implementing AI algorithms in Python. These libraries provide pre-written code and functions that can be easily integrated into your project, saving you time and effort in writing every single line of code from scratch. In this section, we will discuss the most commonly used libraries for AI development in Python and their functionalities.
1. NumPy:
NumPy is a fundamental library for scientific computing with Python. It provides high-performance multidimensional array objects and tools for working with these arrays. This library is widely used for data manipulation, processing, and statistical analysis in AI projects. It also includes linear algebra functions, Fourier transforms, and random number capabilities.
2. Pandas:
Pandas is another essential library for data analysis and manipulation tasks in Python. It offers fast and efficient data structures to work with structured or tabular data such as CSV files or relational databases. With Pandas, you can easily load datasets into memory, clean them up, perform operations like filtering or joining tables, and export the results back to different file formats.
3. Matplotlib:
Matplotlib is a popular visualization library that helps create 2D plots from numerical data in Python. It offers various plotting styles such as line plots, bar charts, scatter plots, histograms among others. With Matplotlib’s extensive customization options, users can add labels, titles or adjust color schemes to make their visualizations more informative and visually appealing.
4. Scikit-learn:
Scikit-learn is an open
– Preparing Data for Training
Preparing Data for Training:
Data is the cornerstone of any artificial intelligence (AI) algorithm. In order to train a model and make accurate predictions, it is crucial to have high-quality and well-structured data. Poor data quality can lead to inaccurate results and ultimately undermine the effectiveness of your AI implementation.
In this section, we will discuss the steps involved in preparing data for training an AI model. These steps are essential for ensuring that your model produces reliable and meaningful results.
1. Define Your Data Requirements:
The first step in preparing data for training is to clearly define your data requirements. This involves understanding what type of data you need, how much data is required, and what format the data should be in. It’s important to be specific about these requirements as they will guide you in selecting the right dataset for your project.
2. Identify Relevant Datasets:
Once you have defined your data requirements, the next step is to identify relevant datasets that contain the necessary information. There are various sources from which you can obtain datasets such as public repositories like Kaggle or UCI Machine Learning Repository, or through web scraping techniques if needed.
3. Cleanse Your Data:
Before using any dataset for training, it is crucial to clean it thoroughly. This process involves identifying and removing any irrelevant or duplicate data points, handling missing values, correcting errors, and standardizing formats if necessary. Cleaning your dataset ensures that there are no biases or inconsistencies present that could affect the performance of your model.
– Choosing and Training an Algorithm
Choosing and training the right algorithm is a crucial step in implementing AI algorithms in Python. It can significantly impact the performance and accuracy of your model. In this section, we will discuss some key points to consider when choosing an algorithm and how to train it effectively.
1. Understand Your Data: The first step in selecting the right algorithm is to understand your data. You need to analyze the type of data you have, its size, structure, and complexity. This will help you identify which type of algorithm would be suitable for your data.
2. Define Your Problem: Before diving into selecting an algorithm, it is essential to define your problem clearly. Different algorithms are designed for specific tasks such as classification, regression, clustering, or recommendation systems. Knowing what you want to achieve with your data will narrow down your options and make it easier to choose the right algorithm.
3. Consider Performance Metrics: Once you have identified the problem and understood your data, think about what metrics are important for evaluating the performance of your model. For example, if you are working on a classification problem, accuracy may be a critical metric while for regression problems; mean squared error (MSE) could be more relevant.
4. Research Different Algorithms: There are various AI algorithms available that can solve different types of problems. Some popular ones include Linear Regression for continuous variables prediction, K-Means Clustering for grouping similar data points together, Decision Trees for classification tasks and Support Vector Machines (SVMs) for both classification
– Evaluating Model Performance
When implementing AI algorithms in Python, evaluating the performance of your models is a crucial step to ensure their effectiveness and accuracy. In this section, we will discuss the various methods and techniques for evaluating model performance.
1. Train-Test Split:
The first method for evaluating model performance is by using a train-test split. This involves dividing your dataset into two subsets – one for training the model and another for testing its performance. The training subset is used to train the model on the data and learn patterns, while the testing subset is used to evaluate its performance on unseen data. By comparing the predicted values from the test set with their actual values, you can determine how well your model performs.
2. Cross-Validation:
Cross-validation is another commonly used technique for evaluating model performance. It involves partitioning your dataset into k folds and then running k separate training and testing iterations. Each time, a different fold is used as the testing set while the rest are used for training. This helps to reduce bias in model evaluation and provides a more accurate estimate of its true performance.
3. Confusion Matrix:
A confusion matrix is a useful tool for evaluating classification models’ performance by visualizing their predictive accuracy on each class in a dataset. It displays four metrics – true positives (TP), false positives (FP), true negatives (TN), and false negatives (FN). These metrics help evaluate how well your model distinguishes between different classes.
Common Challenges and Solutions in Implementing AI:
Implementing AI algorithms can be a challenging task, even for experienced programmers. It requires in-depth knowledge of machine learning techniques, data analysis, and programming languages. In this section, we will outline some common challenges that developers may face when implementing AI algorithms and provide possible solutions to overcome them.
1. Data Collection and Preparation:
One of the most significant challenges in implementing AI is collecting and preparing large datasets for training the algorithm. The quality of data plays a crucial role in the performance of an AI model. Incomplete or biased data can lead to inaccurate predictions or decisions by the algorithm.
Solution: To address this challenge, it is essential to have a thorough understanding of the problem at hand and gather relevant data from reliable sources. Data cleaning techniques such as removing duplicates, handling missing values, and balancing imbalanced datasets can also improve the quality of the data.
2. Choosing the Right Algorithm:
The success of an AI project depends on selecting the right algorithm for a given problem statement. With numerous algorithms available, it can be challenging to determine which one will best suit your needs.
Solution: Start by gaining a general understanding of different machine learning techniques such as supervised learning, unsupervised learning, reinforcement learning, etc., and their use cases. Then evaluate each algorithm’s strengths and weaknesses based on your dataset’s characteristics before deciding on one that aligns with your project goals.
3. Overfitting/Underfitting:
Overfitting occurs when a machine learning model learns the training data too well, capturing noise and random fluctuations rather than the underlying patterns. As a result, the model performs very well on the training data but poorly on unseen data (validation or test data).