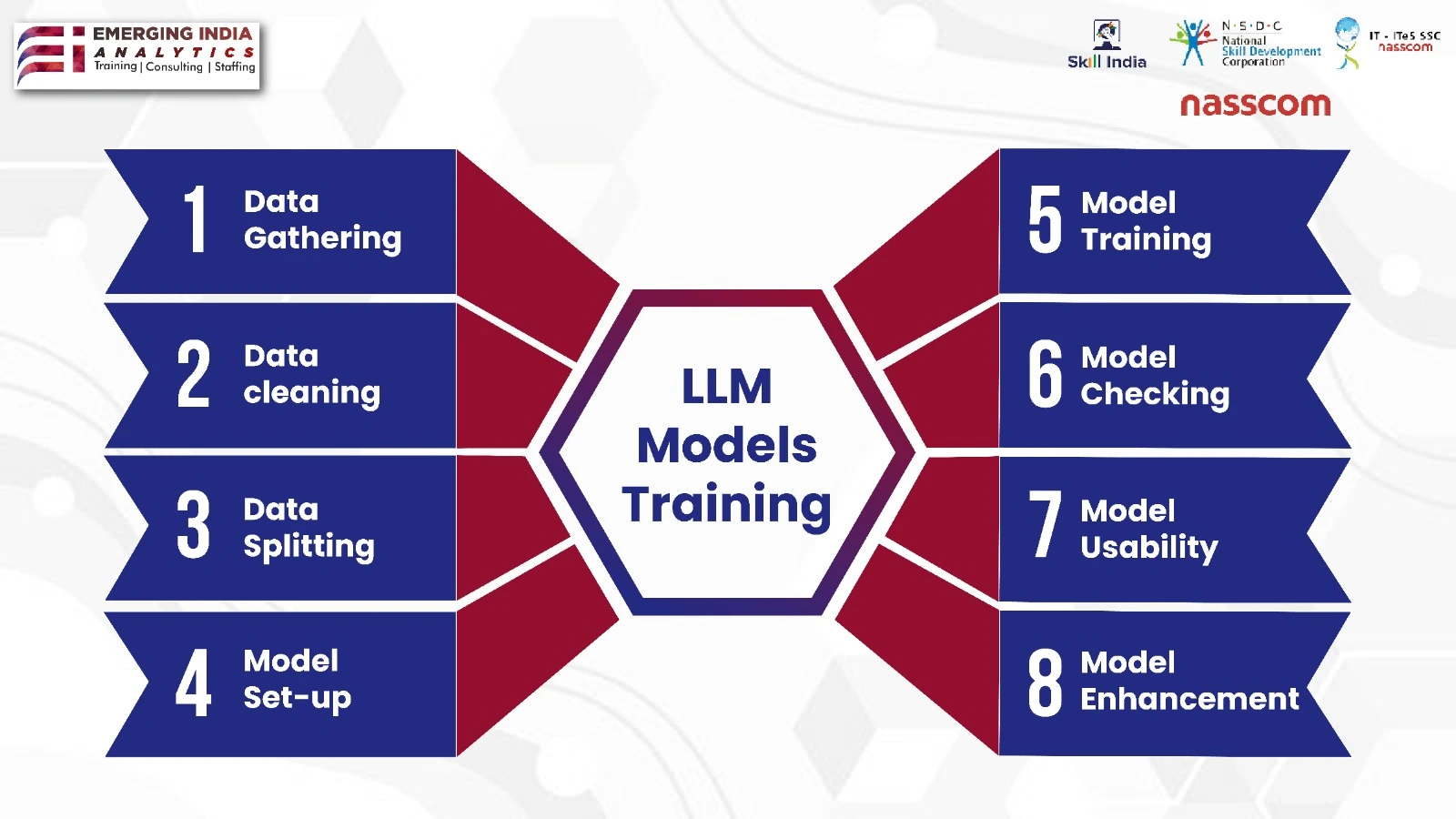
Large Language Models (LLMs) are advanced AI models designed to understand, generate, and manipulate human language. They are typically built using deep learning techniques and trained on massive datasets of text to learn patterns, context, and semantics.
Key Characteristics
Scale: LLMs have a large number of parameters (often in billions), enabling them to capture intricate details of language.
Training Data: Trained on diverse and extensive text data from various sources like books, websites, and articles.
Capabilities
Text Generation: Create coherent and contextually relevant text.
Translation: Translate text between languages.
Summarization: Condense long texts into concise summaries.
Question Answering: Provide accurate answers to user queries.
Conversational Agents: Power chatbots and virtual assistants.
Steps for LLM implementation
Here’s a brief explanation of each step in the process of training and using Large Language Models (LLMs):
1. Data Gathering
Collecting a large and diverse dataset from various sources such as books, websites, articles, and more. This data is crucial for training the LLM to understand different contexts and semantics.
2. Data Cleaning
Processing the gathered data to remove noise, inconsistencies, and irrelevant information. This step ensures that the training data is of high quality, which improves the performance of the LLM.
3. Data Splitting
Dividing the cleaned data into training, validation, and test sets. The training set is used to train the model, the validation set is used to tune hyperparameters and avoid overfitting, and the test set is used to evaluate the model’s performance.
4. Model Training
Using the training data to train the LLM. This involves adjusting the model’s parameters to minimize the difference between the predicted outputs and the actual outputs in the training data.
5. Model Checking
Evaluating the trained model on the validation and test sets to check its performance. This step ensures that the model generalizes well to new, unseen data.
6. Model Usability
Deploying the trained model in real-world applications. This involves integrating the model into systems where it can be used for tasks like text generation, translation, summarization, and more.
7. Model Enhancement
Improving the model based on feedback and new data. This can involve further training, fine-tuning, or incorporating new techniques to enhance the model’s capabilities and performance.
8. Model Enhancement
Continuously monitoring and updating the model to ensure it remains effective and relevant. This includes addressing any issues that arise, such as biases or inaccuracies, and updating the model with new data and techniques.
These steps outline the typical workflow in developing and deploying Large Language Models, ensuring they are accurate, effective, and continuously improving.
Popular Examples
GPT-3 (Generative Pre-trained Transformer 3): Developed by OpenAI, known for its versatility in generating human-like text.
BERT (Bidirectional Encoder Representations from Transformers): Developed by Google, excels in understanding context and semantics.
T5 (Text-To-Text Transfer Transformer): Converts all NLP tasks into a text-to-text format, enhancing its flexibility.
Example of Using GPT-2 with Hugging Face Transformers:
The Hugging Face Transformers library is a powerful tool that provides pre-trained models and tools for natural language processing (NLP) tasks. Here’s an example of how to use the GPT-2 model for text generation:
Installation
First, ensure you have the transformers and torch libraries installed:
pip install transformers torch
Code Example python
from transformers import GPT2LMHeadModel, GPT2Tokenizer
# Load pre-trained model and tokenizer
model_name = ‘gpt2’ # You can also use ‘gpt2-medium’, ‘gpt2-large’, ‘gpt2-xl’ for larger models
tokenizer = GPT2Tokenizer.from_pretrained(model_name)
model = GPT2LMHeadModel.from_pretrained(model_name)
# Encode input text
input_text = “Once upon a time”
input_ids = tokenizer.encode(input_text, return_tensors=’pt’)
# Generate text
output = model.generate(input_ids, max_length=100, num_return_sequences=1)
# Decode generated text
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
print(generated_text)
Explanation
Import Libraries:
python
from transformers import GPT2LMHeadModel, GPT2Tokenizer
This line imports the necessary classes from the transformers library. GPT2LMHeadModel is the GPT-2 model class, and GPT2Tokenizer is the tokenizer class used to convert text to token IDs and back.
Load Pre-trained Model and Tokenizer:
Python
model_name = ‘gpt2’
tokenizer = GPT2Tokenizer.from_pretrained(model_name)
model = GPT2LMHeadModel.from_pretrained(model_name)
Here, we specify the model name (gpt2) and load the pre-trained model and tokenizer using the from_pretrained method. This downloads the model and tokenizer if not already cached.
Encode Input Text:
python
input_text = “Once upon a time”
input_ids = tokenizer.encode(input_text, return_tensors=’pt’)
The input text is encoded into token IDs using the tokenizer. The return_tensors=’pt’ argument ensures that the output is a PyTorch tensor, which is required for the model.
Generate Text:
python
output = model.generate(input_ids, max_length=100, num_return_sequences=1)
This line generates text from the input token IDs. The max_length parameter specifies the maximum length of the generated text, and num_return_sequences specifies the number of different sequences to generate.
Decode Generated Text:
python
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
The generated token IDs are decoded back into text using the tokenizer. The skip_special_tokens=True argument removes special tokens like <|endoftext|> from the output.
Print Generated Text:
python
print(generated_text)
Finally, the generated text is printed to the console.
Output
When you run the above code, you might get an output like this:
“Once upon a time, there was a little girl named Lily who lived in a small village nestled in the mountains. Every day, she would venture into the forest to gather berries and play with her animal friends. One day, while exploring a new path, she stumbled upon a hidden cave. Inside the cave, she discovered a magical treasure that glowed with a mysterious light. As she reached out to touch it, she was transported to a fantastical world filled with dragons, wizards, and enchanted forests. Lily’s adventure had just begun, and she knew that she would never be the same again.”
Note that the exact output may vary each time you run the code due to the probabilistic nature of the model’s text generation process.
LLMs represent a significant leap in AI’s ability to understand and generate human language, offering numerous possibilities across various fields while also posing challenges that need careful consideration.
Applications
- Content Creation: Writing articles, stories, and marketing copy.
- Customer Support: Automating responses to customer queries.
- Research Assistance: Summarizing research papers and extracting key information.
- Education: Providing tutoring and answering educational questions.
- Healthcare: Assisting in medical documentation and patient interaction.
Challenges
- Bias: LLMs can perpetuate biases present in training data.
- Interpretability: Understanding how LLMs make decisions can be difficult.
- Resource Intensive: Training and deploying LLMs require significant computational resources.
- Ethical Concerns: Misuse for generating fake news, spam, or malicious content.
Future Directions
- Improved Efficiency: Developing models that are less resource-intensive.
- Ethical AI: Implementing measures to reduce bias and ensure ethical use.
- Domain-Specific Models: Training LLMs on specialized datasets for industry-specific applications.
- Enhanced Understanding: Making models better at reasoning and understanding complex queries.
LLMs represent a significant leap in AI’s ability to understand and generate human language, offering numerous possibilities across various fields while also posing challenges that need careful consideration.