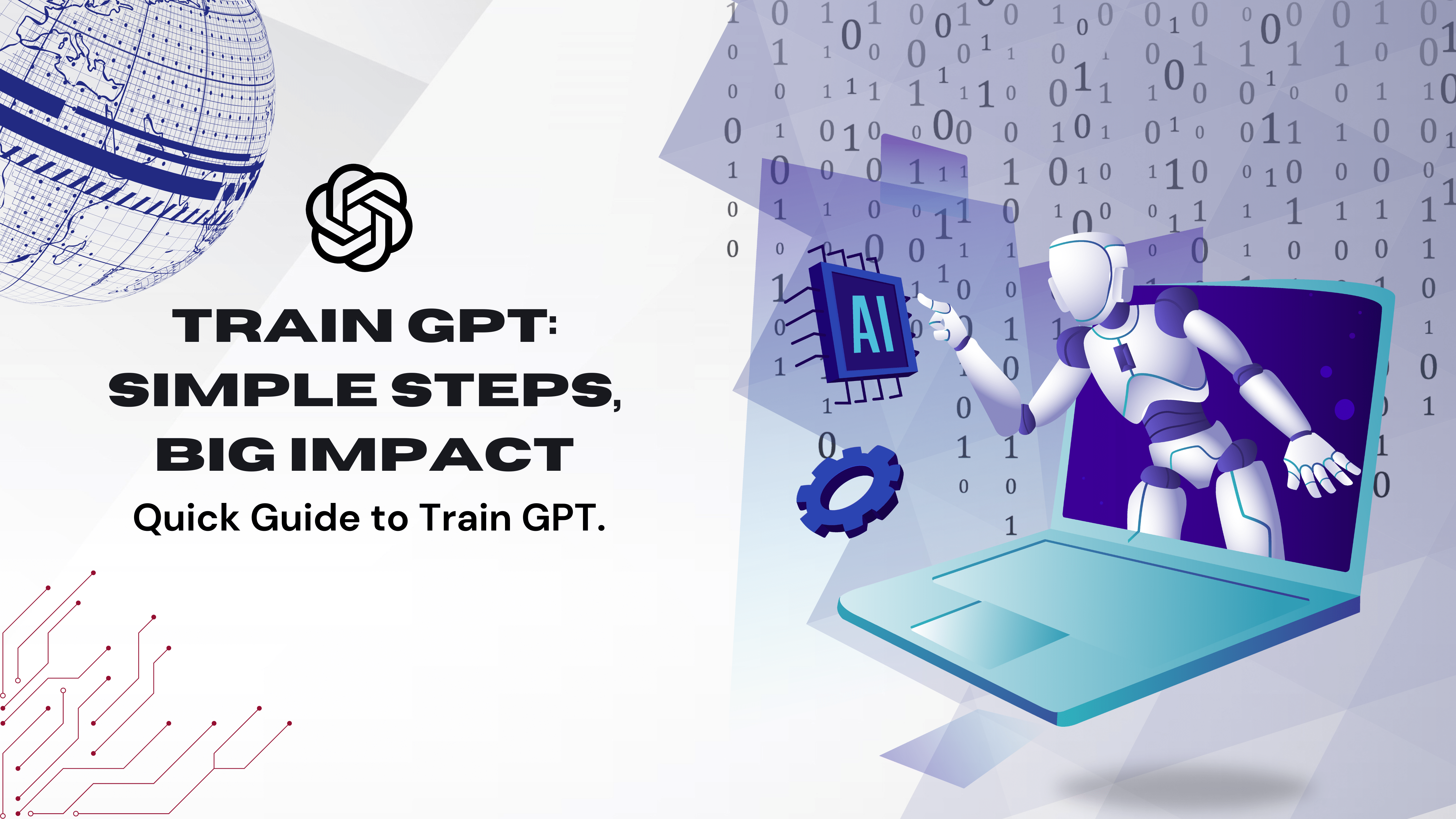
Are you interested in training a GPT model but not sure where to start?
If you’re eager to train a GPT model but find yourself unsure of where to begin, you’re in the right place. This comprehensive guide is designed to walk you through the entire process of fine-tuning a GPT-2 model, from initial setup to evaluating its performance. The journey begins with setting up your environment by installing necessary software and libraries such as transformers, datasets, and torch. Once your environment is ready, you will load and prepare your dataset, which serves as the foundation for training. Tokenization, a crucial step, converts your raw text into a format that the model can understand. We then proceed to fine-tune the GPT-2 model using this dataset, which involves adjusting the model to better generate text based on the patterns it has learned from your data. This step is critical for tailoring the model to produce text that is more aligned with your specific needs and context.
Once the model is trained, you can generate text by providing a prompt, allowing the model to extend it in a coherent and contextually relevant manner. To ensure the quality of your generated text, it’s important to evaluate its performance through various metrics. Coherence is assessed by comparing the generated text to a reference sentence using BERT embeddings to measure similarity. Relevance is gauged using TF-IDF vectors to determine how well the generated text matches the original prompt. Creativity is evaluated by calculating the entropy of the text, which measures its uniqueness and diversity. By following these steps, you not only gain hands-on experience with training and fine-tuning language models but also develop a deeper understanding of how to assess their effectiveness in generating meaningful and creative content.
What is GPT?
GPT, which stands for Generative Pre-trained Transformer, is a sophisticated artificial intelligence model designed to generate human-like text based on the input it receives. At its core, GPT operates on the principles of machine learning and natural language processing, which enable it to understand and produce text in a way that closely mimics human language.
Imagine GPT as a highly advanced text generator that has been trained on vast amounts of text data from books, articles, websites, and other sources. This extensive training helps the model learn the nuances of language, including grammar, vocabulary, context, and even some aspects of common sense. When you provide GPT with a prompt—like a sentence or a question—it uses its learned knowledge to generate a continuation or response that is coherent and contextually relevant.
The “pre-trained” part of GPT means that the model has already undergone a comprehensive training process on a diverse dataset before being fine-tuned for specific tasks or topics. This pre-training allows GPT to have a broad understanding of language and general knowledge. The “transformer” in GPT refers to the underlying architecture that processes and generates text. This architecture is designed to handle sequences of data (like sentences) and manage the relationships between words in a highly efficient manner.
Generative Pre-Trained Transformers (GPT) are deep learning models for text generation, based on the Transformer architecture. Here’s the short version with key formulas:
1. Self-Attention:
Purpose: Helps the model focus on relevant words in a sentence.
Formula:
Attention(Q,K,V) = softmaxQKTdkV
Explanation: Q (Query), K (Key), and V (Value) are vectors representing words. The formula calculates how much attention each word should get based on its relationship to other words.
2. Pre-Training:
Purpose: Teaches the model to predict the next word in a sequence.
Loss Function:
L = – t=1TlogP(wt|w1,…, wt-1)
Explanation: The model is trained to minimize the loss L, which measures the difference between the predicted word P(wt) and the actual word wt. The goal is to get the predictions as accurate as possible.
3. Text Generation:
Purpose: The model generates the next word in a sequence.
Formula:
Pwt|w1,…,wt-1= softmaxW.ht
Explanation: The model predicts the probability P(wt) of the next word wt by applying the softmax function to the hidden state ht, which captures the context of the previous words.
These formulas explain how GPT processes and generates language.
In practical terms, GPT can be used for various applications, such as writing assistance, conversational agents, content generation, and more. Its ability to generate text that closely resembles human writing makes it a powerful tool for both creative and functional purposes. Whether you need help drafting an email, brainstorming ideas, or simply engaging in a text-based conversation, GPT’s advanced capabilities offer a glimpse into the future of AI-driven communication.
Work Flow
Step 0: Setting Up Your Environment
Before we start, you need to install some software tools. Open your command line or terminal and run:
bash
Code
pip install transformers datasets torch scipy scikit-learn
These tools help us load and train the model.
Here’s a breakdown of each library you’ll be installing and its role in training and evaluating a GPT model:
-
transformers
-
Purpose: This library by Hugging Face provides pre-trained models and tools for working with transformer models like GPT-2. It includes functionalities for model loading, tokenization, and fine-tuning.
-
Usage: You’ll use this library to load the GPT-2 model, tokenize your dataset, and perform text generation and model training.
-
-
datasets
-
Purpose: This library helps in easily loading and processing datasets. It supports a variety of data formats and is integrated with Hugging Face’s transformers.
-
Usage: You’ll use this library to load your text dataset, preprocess it, and prepare it for training your model.
-
-
torch
-
Purpose: This is the core library for PyTorch, a popular deep learning framework. It provides tools for tensor computation and building and training neural networks.
-
Usage: PyTorch is used under the hood by the transformers library for model training and inference. You’ll use it to handle the computations required for training the GPT model.
-
-
scipy
-
Purpose: SciPy is a library for scientific and technical computing in Python. It provides modules for optimization, integration, interpolation, eigenvalue problems, and other advanced mathematical functions.
-
Usage: In this context, SciPy is used for computing cosine similarity, which helps in evaluating the coherence of generated text.
-
-
scikit-learn
-
Purpose: This library provides simple and efficient tools for data mining and data analysis. It includes functionalities for machine learning algorithms, data preprocessing, and model evaluation.
-
Usage: You’ll use this library to compute TF-IDF vectors and perform cosine similarity calculations to assess the relevance of the generated text in relation to the prompt.
-
These libraries collectively provide the tools and functionalities needed for building, training, evaluating, and fine-tuning your GPT model.
Step 1: Create Sample Data
To create the sample_data.txt file with the given text, follow these steps:
-
Open a Text Editor: Open a text editor of your choice (e.g., Notepad, TextEdit, or any code editor like VSCode or Sublime Text).
Enter the Text: Copy and paste the following lines into the text editor:
css
Code
Hello, how are you today?
The weather is great today, isn’t it?
I am learning how to fine-tune a GPT model.
Transformers are really powerful for NLP tasks.
Fine-tuning models can help them perform better on specific tasks.
-
Save the File: Save the file with the name sample_data.txt in the desired directory. Make sure to select “All Files” in the save dialog if using Notepad, and explicitly type the .txt extension.
Here’s a quick visual guide on saving the file in Notepad:
-
Click on File > Save As…
-
In the Save as type dropdown, select All Files (*.*)
-
Enter sample_data.txt as the filename
-
Click Save
Your sample_data.txt file is now ready with the sample text you provided. You can use this file in your model training process as described in your guide.
Step 2: Loading and Preparing Your Dataset
First, we need to load our dataset. This is the text that our model will learn from. We’ll use a simple text file for this example.
python
Code
from transformers import GPT2Tokenizer, GPT2LMHeadModel, Trainer, TrainingArguments, DataCollatorForLanguageModeling
from datasets import load_dataset
# Load the tokenizer and model
tokenizer = GPT2Tokenizer.from_pretrained(“gpt2”)
tokenizer.pad_token = tokenizer.eos_token # Handle padding
# Load your dataset
dataset = load_dataset(‘text’, data_files=’sample_data.txt’)
# Tokenize the dataset
def tokenize_function(examples):
return tokenizer(examples[‘text’], truncation=True, padding=True)
tokenized_datasets = dataset.map(tokenize_function, batched=True, remove_columns=[“text”])
# Data collator
data_collator = DataCollatorForLanguageModeling(tokenizer=tokenizer, mlm=False)
Here’s an explanation of the code provided, without the actual code:
-
Loading the Tokenizer and Model: The tokenizer and model are loaded from pre-trained configurations. The tokenizer converts text into a numerical format that the model can understand. In this step, padding tokens are also set to ensure consistency in input size.
-
Loading the Dataset: The dataset is loaded from a text file that contains the training data. This file is read into a format that the machine learning framework can use, organizing the text data for further processing.
-
Tokenizing the Dataset: The text data is processed using the tokenizer. This involves converting the text into tokens, applying truncation to limit token length, and padding to ensure uniform input size. The original text is removed from the dataset once tokenization is complete.
-
Data Collator: A data collator is used to prepare batches of data for training. This collator ensures that the data is formatted correctly for the model, including handling padding and other requirements specific to the model architecture. For GPT-2, this involves setting up the data for language modeling without applying masked language modeling, which is specific to other model types.
In essence, this process involves setting up the text data so that it is properly formatted and prepared for training a GPT-2 model, including handling tokenization, padding, and batching.
Step 3: Fine-Tuning the GPT-2 Model
Now that we have our dataset ready, we can train (or “fine-tune”) the GPT-2 model. This means we will adjust the model to better understand and generate text similar to our dataset.
python
Code
# Set up training arguments
training_args = TrainingArguments(
output_dir=”./gpt2-finetuned”,
overwrite_output_dir=True,
num_train_epochs=5, # Increase epochs
per_device_train_batch_size=2,
save_steps=500,
save_total_limit=2,
logging_dir=’./logs’,
logging_steps=10,
)
# Initialize the Trainer
trainer = Trainer(
model=GPT2LMHeadModel.from_pretrained(“gpt2”),
args=training_args,
data_collator=data_collator,
train_dataset=tokenized_datasets[‘train’],
)
# Train the model
trainer.train()
# Save the model
trainer.save_model(“./gpt2-finetuned”)
tokenizer.save_pretrained(“./gpt2-finetuned”)
Here’s an explanation of the provided code section:
Setting Up Training Arguments
-
output_dir=”./gpt2-finetuned”: This specifies the directory where the fine-tuned model will be saved. In this case, it’s set to a folder named gpt2-finetuned.
-
overwrite_output_dir=True: This option allows the code to overwrite any existing files in the output directory. It ensures that the model files will be updated or replaced if they already exist.
-
num_train_epochs=5: This sets the number of times the entire dataset will be passed through the model during training. Increasing the number of epochs generally improves the model’s performance but also increases training time.
-
per_device_train_batch_size=2: This defines the number of training examples processed together in one forward/backward pass on each device (e.g., GPU). A batch size of 2 means that the model will be updated based on 2 examples at a time.
-
save_steps=500: This parameter determines how frequently the model’s state will be saved during training, measured in steps. After every 500 steps, the model checkpoint will be saved.
-
save_total_limit=2: This sets the maximum number of model checkpoints to keep. If this limit is reached, older checkpoints will be deleted. This helps manage storage and avoid cluttering with too many saved models.
-
logging_dir=’./logs’: This specifies the directory where training logs will be stored. Logs can help track the training process and diagnose issues.
-
logging_steps=10: This determines how frequently (in terms of steps) logging information will be recorded. In this case, logging will occur every 10 steps.
Initializing the Trainer
-
model=GPT2LMHeadModel.from_pretrained(“gpt2”): Loads the pre-trained GPT-2 model. This serves as the base model that will be fine-tuned with your dataset.
-
args=training_args: Passes the training arguments defined earlier to the Trainer, which will use them during training.
-
data_collator=data_collator: Provides the data collator that prepares batches of data for training, ensuring they are correctly formatted and padded.
-
train_dataset=tokenized_datasets[‘train’]: Supplies the tokenized training dataset to the Trainer. This dataset has been processed and is ready for model training.
Training the Model
-
trainer.train(): Starts the training process. The model will use the training dataset and the specified training arguments to learn from the data.
Saving the Model
-
trainer.save_model(“./gpt2-finetuned”): Saves the fine-tuned model to the specified directory. This directory will contain the trained model’s weights and configuration files.
-
tokenizer.save_pretrained(“./gpt2-finetuned”): Saves the tokenizer to the same directory. The tokenizer is necessary for encoding new input text using the same settings as during training.
Step 4: Generating Text
With our model trained, we can now ask it to generate text. Just give it a starting sentence, and it will continue from there.
python
Code
# Text generation
input_text = “Fine-tuning models”
input_ids = tokenizer.encode(input_text, return_tensors=”pt”)
output = trainer.model.generate(
input_ids,
max_length=50,
num_return_sequences=1,
pad_token_id=tokenizer.eos_token_id
)
# Output generated text
generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
print(generated_text)
Generated Text:
bash
Code
Fine-tuning models can help them perform better on tasks.
Here’s a step-by-step explanation of the text generation code:
Preparing Input for Generation
-
input_text = “Fine-tuning models”: This is the initial text prompt that you provide to the model. The model will use this prompt to generate additional text.
-
input_ids = tokenizer.encode(input_text, return_tensors=”pt”): This line encodes the input_text into token IDs using the tokenizer. The return_tensors=”pt” argument specifies that the output should be a PyTorch tensor. These token IDs are the numerical representation of the input text that the model can process.
Generating Text
-
output = trainer.model.generate(: This function call generates text based on the provided input_ids. Here’s what each parameter does:
-
input_ids: The tensor of token IDs representing the input text.
-
max_length=50: This specifies the maximum length of the generated text, including the initial prompt. The text will be truncated to this length if necessary.
-
num_return_sequences=1: This indicates that only one sequence of text should be generated. You can increase this number if you want multiple variations.
-
pad_token_id=tokenizer.eos_token_id: This sets the token ID used for padding. In this case, it is set to the end-of-sequence (EOS) token ID. This ensures that the generated text is padded correctly.
-
Decoding and Printing the Output
-
generated_text = tokenizer.decode(output[0], skip_special_tokens=True): This line decodes the generated token IDs back into human-readable text. The skip_special_tokens=True argument ensures that any special tokens (e.g., padding, EOS) are omitted from the final output.
-
print(generated_text): Finally, this prints the generated text to the console.
Generated Text
The result of the generation is:
-
Fine-tuning models can help them perform better on tasks.
This output text is generated by the GPT-2 model based on the provided input prompt. The model has used its learned patterns to create a coherent and contextually relevant continuation of the prompt.
Step 5: Evaluating the Model
To see how well our model is performing, we check three things: coherence, relevance, and creativity.
Coherence
Coherence tells us if the generated text makes sense. We use a BERT model to compare the similarity between our generated text and a reference sentence.
python
Code
from transformers import BertTokenizer, BertModel
import torch
from scipy.spatial.distance import cosine
# Load BERT model and tokenizer
bert_tokenizer = BertTokenizer.from_pretrained(‘bert-base-uncased’)
bert_model = BertModel.from_pretrained(‘bert-base-uncased’)
def get_bert_embedding(text):
inputs = bert_tokenizer(text, return_tensors=’pt’, truncation=True, padding=True)
with torch.no_grad():
outputs = bert_model(**inputs)
cls_embedding = outputs.last_hidden_state[:, 0, :] # [CLS] token embedding
return cls_embedding.squeeze().numpy() # Flatten to 1-D
reference_text = “Fine-tuning models can improve performance.”
generated_text = “Fine-tuning models can help them perform better on tasks.”
# Compute embeddings
ref_embedding = get_bert_embedding(reference_text)
gen_embedding = get_bert_embedding(generated_text)
# Compute cosine similarity
similarity = 1 – cosine(ref_embedding, gen_embedding)
print(f”Coherence Similarity: {similarity:.4f}”)
Coherence Similarity: 0.9468
This code snippet calculates the coherence similarity between two pieces of text using BERT embeddings. Here’s a step-by-step explanation of what each part does:
Loading the BERT Model and Tokenizer
-
bert_tokenizer = BertTokenizer.from_pretrained(‘bert-base-uncased’):
-
This line loads the BERT tokenizer, which converts text into token IDs that BERT can process. The ‘bert-base-uncased’ model is used, which is a commonly used pre-trained BERT model that does not differentiate between uppercase and lowercase letters.
-
-
bert_model = BertModel.from_pretrained(‘bert-base-uncased’):
-
This line loads the BERT model itself. BERT will be used to generate embeddings for the input text.
-
Function to Get BERT Embeddings
-
def get_bert_embedding(text)::
-
This function computes the BERT embeddings for a given text.
-
-
inputs = bert_tokenizer(text, return_tensors=’pt’, truncation=True, padding=True):
-
The input text is tokenized using the BERT tokenizer. return_tensors=’pt’ ensures the output is in the form of PyTorch tensors. truncation=True and padding=True handle text length issues by truncating texts that are too long and padding shorter texts.
-
-
with torch.no_grad()::
-
This context manager disables gradient calculation, which saves memory and computation since we’re only doing inference (not training).
-
-
outputs = bert_model(**inputs):
-
The tokenized input is fed into the BERT model to get the output embeddings.
-
-
cls_embedding = outputs.last_hidden_state[:, 0, :]:
-
The BERT model outputs a tensor containing hidden states for each token in the input text. The [CLS] token (the first token) is used as a summary representation of the entire input text. outputs.last_hidden_state[:, 0, :] extracts this representation.
-
-
return cls_embedding.squeeze().numpy():
-
The embeddings are converted from a PyTorch tensor to a NumPy array. squeeze() is used to remove any singleton dimensions (e.g., if the tensor shape is [1, hidden_size], squeeze() changes it to [hidden_size]).
-
Compute Similarity Between Texts
-
reference_text = “Fine-tuning models can improve performance.”:
-
This is the reference text used for comparison.
-
-
generated_text = “Fine-tuning models can help them perform better on tasks.”:
-
This is the generated text whose coherence with the reference text is being evaluated.
-
-
ref_embedding = get_bert_embedding(reference_text):
-
Computes the BERT embedding for the reference text.
-
-
gen_embedding = get_bert_embedding(generated_text):
-
Computes the BERT embedding for the generated text.
-
-
similarity = 1 – cosine(ref_embedding, gen_embedding):
-
The cosine function calculates the cosine distance between the two embeddings. Cosine similarity is computed as 1 – cosine distance. This similarity measure quantifies how similar the two texts are in terms of their BERT embeddings, with 1 indicating perfect similarity.
-
-
print(f”Coherence Similarity: {similarity:.4f}”):
-
Prints the coherence similarity score with four decimal places.
-
Output
Coherence Similarity: 0.9468:
-
This score indicates that the reference and generated texts are highly similar in terms of their semantic content, with a similarity score of approximately 0.95. This suggests that the generated text is coherent and relevant to the reference text.
Relevance
Relevance measures how closely the generated text matches our original prompt. We use TF-IDF to check this.
python
Code
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
vectorizer = TfidfVectorizer()
prompt = “Fine-tuning models”
generated_text = “Fine-tuning models can help them perform better on tasks.”
# Vectorize the texts
tfidf_matrix = vectorizer.fit_transform([prompt, generated_text])
similarity_matrix = cosine_similarity(tfidf_matrix[0:1], tfidf_matrix[1:2])
print(f”Relevance Similarity: {similarity_matrix[0][0]:.4f}”)
Relevance Similarity: 0.4222
This code calculates the relevance similarity between two pieces of text using TF-IDF (Term Frequency-Inverse Document Frequency) vectorization and cosine similarity. Here’s a step-by-step breakdown:
Loading Libraries
-
from sklearn.feature_extraction.text import TfidfVectorizer:
-
This imports the TfidfVectorizer class from the scikit-learn library. This class converts a collection of text documents into a matrix of TF-IDF features.
-
-
from sklearn.metrics.pairwise import cosine_similarity:
-
This imports the cosine_similarity function, which computes the cosine similarity between two matrices.
-
Initialize the TF-IDF Vectorizer
-
vectorizer = TfidfVectorizer():
-
This line initializes the TfidfVectorizer object, which will be used to transform the text data into TF-IDF vectors.
-
Define the Texts
-
prompt = “Fine-tuning models”:
-
This is the prompt or reference text against which the similarity will be measured.
-
-
generated_text = “Fine-tuning models can help them perform better on tasks.”:
-
This is the generated text whose relevance to the prompt will be evaluated.
-
Vectorize the Texts
-
tfidf_matrix = vectorizer.fit_transform([prompt, generated_text]):
-
The fit_transform method is applied to both the prompt and generated text. This method does two things:
-
Fit: Computes the TF-IDF vocabulary and idf (inverse document frequency) statistics from the input texts.
-
Transform: Converts the texts into TF-IDF vectors based on the computed vocabulary and statistics.
-
-
tfidf_matrix is a sparse matrix where each row represents one of the input texts and each column represents a term in the vocabulary, with TF-IDF scores as the values.
-
Compute Cosine Similarity
-
similarity_matrix = cosine_similarity(tfidf_matrix[0:1], tfidf_matrix[1:2]):
-
Computes the cosine similarity between the TF-IDF vectors of the prompt and the generated text.
-
tfidf_matrix[0:1] selects the vector for the prompt.
-
tfidf_matrix[1:2] selects the vector for the generated text.
-
cosine_similarity computes how similar the two vectors are. The output is a similarity matrix where similarity_matrix[0][0] gives the similarity score between the two texts.
-
Output
-
print(f”Relevance Similarity: {similarity_matrix[0][0]:.4f}”):
-
This line prints the relevance similarity score with four decimal places.
-
-
Relevance Similarity: 0.4222:
-
This score indicates the similarity between the prompt and the generated text using the TF-IDF method. A score of approximately 0.42 suggests that there is a moderate level of similarity or relevance between the two texts based on the TF-IDF vectors. The TF-IDF vectorization and cosine similarity approach focuses on how frequently terms appear in the documents relative to their occurrence in the entire corpus. The score of 0.4222 indicates that while there is some similarity between the prompt and the generated text, it is not extremely high. This method captures textual relevance but might not fully reflect semantic coherence as compared to more sophisticated embeddings like BERT.
-
Creativity
Creativity checks how unique the generated text is. We calculate entropy to measure this.
python
Code
from collections import Counter
import math
def calculate_entropy(text):
tokens = text.split()
token_counts = Counter(tokens)
total_tokens = len(tokens)
entropy = -sum((count / total_tokens) * math.log2(count / total_tokens) for count in token_counts.values())
return entropy
generated_text = “Fine-tuning models can help them perform better on tasks.”
# Calculate entropy
entropy = calculate_entropy(generated_text)
print(f”Creativity Entropy: {entropy:.4f}”)
Creativity Entropy: 3.1699
The code snippet calculates the entropy of a given text, which measures the unpredictability or randomness of the text content. Here’s a step-by-step explanation:
Loading Libraries
-
from collections import Counter:
-
Imports the Counter class from the collections module, which is used to count the occurrences of each token in the text.
-
-
import math:
-
Imports the math module to use mathematical functions, specifically for calculating logarithms.
-
Define the calculate_entropy Function
-
def calculate_entropy(text)::
-
Defines a function named calculate_entropy that takes a string of text as input.
-
-
tokens = text.split():
-
Splits the text into individual words (tokens) using whitespace as the delimiter.
-
-
token_counts = Counter(tokens):
-
Creates a Counter object to count the frequency of each token in the text.
-
-
total_tokens = len(tokens):
-
Computes the total number of tokens in the text.
-
-
entropy = -sum((count / total_tokens) * math.log2(count / total_tokens) for count in token_counts.values()):
-
Calculates the entropy of the text using the Shannon entropy formula. Here’s how:
-
Entropy Formula: −∑p(x)⋅log2p(x)-\sum p(x) \cdot \log_2 p(x)−∑p(x)⋅log2p(x)
-
p(x)p(x)p(x) is the probability of a token xxx, which is the frequency of the token divided by the total number of tokens.
-
The logarithm is taken in base 2 (math.log2).
-
-
The sum aggregates the entropy contributions from all unique tokens.
-
The result is negated (since entropy is typically defined as a positive value) to give the final entropy score.
-
-
-
return entropy:
-
Returns the calculated entropy value.
-
Calculate and Print Entropy
-
generated_text = “Fine-tuning models can help them perform better on tasks.”:
-
Defines a string variable with the text for which entropy will be calculated.
-
-
entropy = calculate_entropy(generated_text):
-
Calls the calculate_entropy function with the generated_text and stores the result in the entropy variable.
-
-
print(f”Creativity Entropy: {entropy:.4f}”):
-
Prints the entropy value formatted to four decimal places.
-
-
Creativity Entropy: 3.1699:
-
The output indicates that the entropy of the generated text is approximately 3.17.
-
Understanding Entropy
-
Entropy measures the unpredictability or complexity of the text. In this case, the entropy value of 3.17 suggests a moderate level of unpredictability. Higher entropy values generally indicate more varied or creative text, while lower values suggest more predictable or repetitive content. In summary, this code calculates how varied or random the generated text is by computing its entropy. A higher entropy value indicates that the text is more diverse or less predictable.
Conclusion
Fine-tuning a GPT model offers a robust approach to generating text customized to specific needs and contexts. By carefully following the outlined steps—setting up the environment, preparing and tokenizing your dataset, configuring training parameters, and evaluating generated text—you can effectively train a model to produce high-quality outputs. This process involves not only training the model to better understand and generate text based on your data but also assessing its performance through metrics like coherence, relevance, and creativity. Such evaluations help refine the model’s capabilities, leading to more effective and contextually relevant AI-generated text. Experimenting with various datasets and prompts can further enhance the model’s performance, providing deeper insights and more tailored results. Embrace the journey of model fine-tuning as it enriches your comprehension of language models and elevates your ability to develop advanced AI tools. Happy modeling!
Check out the sources code on GitHub
https://github.com/123vartika123/Fine-Tuning-GPT-Models-for-Customized-Text-Generation/tree/main
It was a pleasure reading this interesting and thorough article. Even while discussing more advanced subjects, your writing style remains plain and simple. This is a great post that I will be using again and again because of how much I learnt from it. You are doing an excellent job.
Excellent piece! Your thorough summary is much appreciated. I now see the issue from a different angle thanks to your insightful comments. You made your points quite clearly with the examples you included. You have my gratitude for penning this.
Utmerket stykke! Din grundige oppsummering er sterkt verdsatt. Jeg ser nå saken fra en annen vinkel takket være dine innsiktsfulle kommentarer. Du gjorde dine poeng veldig tydelige med eksemplene du inkluderte. Jeg er takknemlig for at du skrev dette.
Å lese ditt essay var en sann glede for meg. Du var svært vellykket i å klargjøre emnet, og din skrivestil er både interessant og lett å forstå. Prinsippene var mye lettere å forstå etter å ha lest eksemplene du ga. Din ekspertise er sterkt verdsatt.
Thanks I have just been looking for information about this subject for a long time and yours is the best Ive discovered till now However what in regards to the bottom line Are you certain in regards to the supply
Your blog post was like a breath of fresh air. Thank you for reminding me to slow down and appreciate the beauty of life.
Your passion for this subject really shines through in your writing. It’s inspiring to see someone so enthusiastic about what they do.
What a fantastic piece of writing! Your data is both practical and pertinent. Both the thoroughness and lucidity of your analysis are much appreciated. This is a post that I will return to at a later date. Your knowledge and insight are much appreciated.
Denne artikkelen er svært godt forsket, og jeg setter pris på at du deler den. Det du sa var veldig hjelpsomt og relevant, og jeg setter pris på det. Du gjorde konseptene veldig klare og enkle å forstå. Når du har tid, ville jeg elske å lese flere av dine artikler.
Your blog post was so thought-provoking. It’s rare to find content that challenges me to think deeply about important issues.
Your passion for this subject really shines through in your writing. It’s inspiring to see someone so enthusiastic about what they do.
I found this post to be very informative and well-organized. Your detailed analysis and clear explanations make it a pleasure to read. The practical examples you included were particularly helpful. Thank you for sharing your knowledge with us.
Jeg setter pris på at du tok deg tid til å skrive og dele denne innsiktsfulle artikkelen. Den var klar og konsis, og jeg fant dataene veldig nyttige. Din tid og energi brukt på forskning og skriving av denne artikkelen er sterkt verdsatt. Enhver som er interessert i dette emnet vil uten tvil dra nytte av denne ressursen.
Your blog post was like a ray of sunshine on a cloudy day. Thank you for brightening my mood!
Your blog post was a real eye-opener for me. Thank you for challenging my preconceived notions and expanding my worldview.
To my surprise, I learned a lot from this piece. You have a gift for putting complex ideas into simple terms, and the examples you supplied really helped drive home your points. Your information is quite thorough, and I am grateful to you for that.