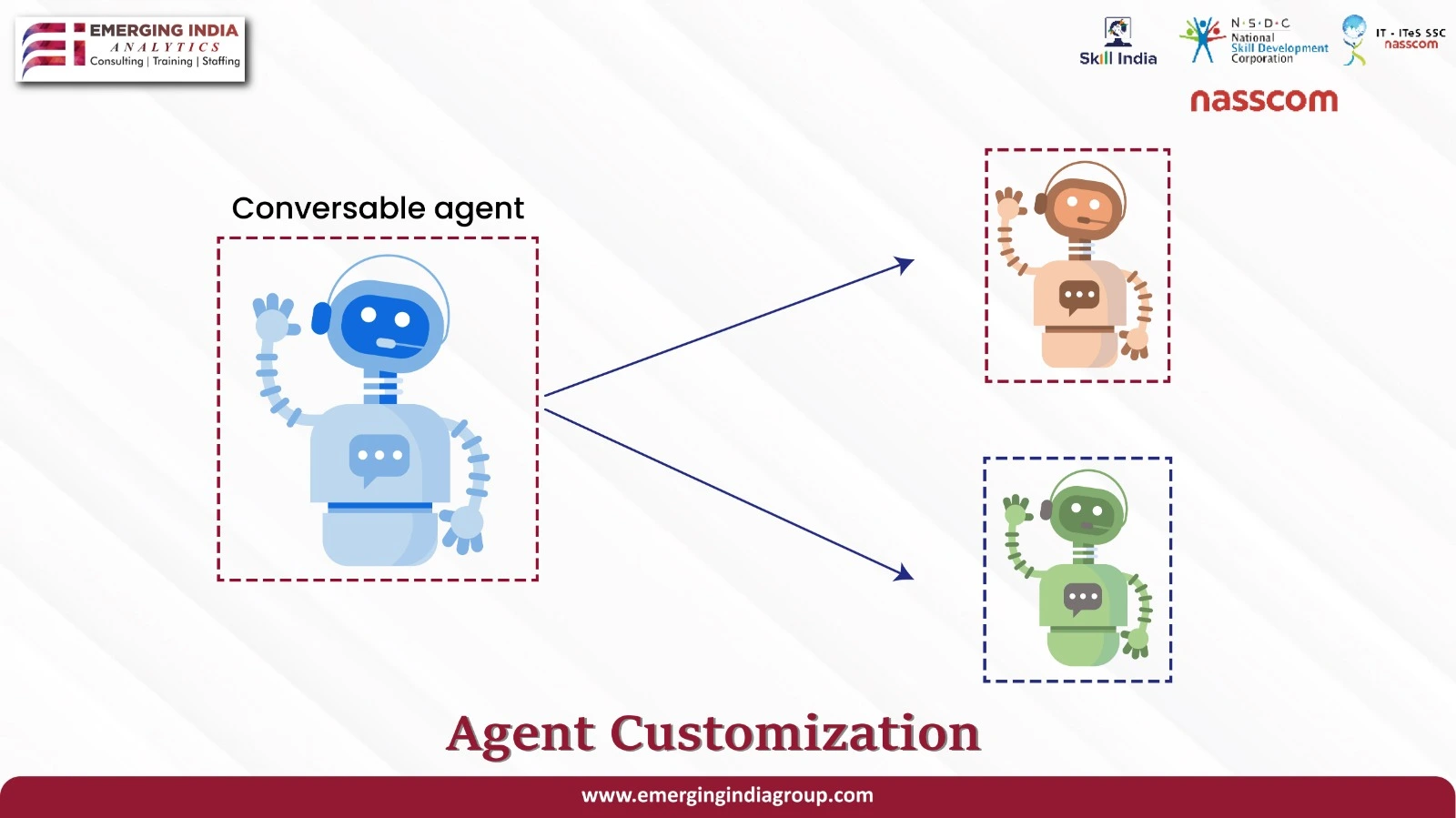
Welcome to the AutoGen Implementation Guide, your gateway to harnessing the transformative power of Large Language Models (LLMs) in automating and enhancing various tasks. Developed at the intersection of cutting-edge AI research from OpenAI and practical implementation through Hugging Face’s transformers library, AutoGen represents a significant leap forward in enabling developers to create intelligent agents capable of handling complex workflows and interactions.
In today’s rapidly evolving technological landscape, the demand for efficient automation tools has never been higher. AutoGen stands out as a versatile solution designed to leverage the capabilities of LLMs to automate tasks that traditionally require human intervention. Whether it’s generating human-like text, simulating conversations, or executing complex workflows, AutoGen empowers developers to integrate advanced AI functionalities seamlessly into their applications.
Understanding OpenAI: Pioneers in Safe and Beneficial AI
OpenAI, renowned for its commitment to advancing safe and beneficial artificial intelligence, has revolutionized the field with breakthroughs like GPT-3 and GPT-4. These models are celebrated for their ability to understand and generate human-like text, making them indispensable for applications ranging from chatbots and content creation to language translation and beyond. By leveraging OpenAI’s advancements, AutoGen ensures that developers have access to state-of-the-art AI capabilities that are both powerful and ethically grounded.
Hugging Face’s Transformers Library: Empowering NLP Innovation
At the heart of AutoGen lies Hugging Face’s transformers library, a cornerstone of modern natural language processing (NLP). This open-source toolkit provides developers with access to pre-trained models such as BERT, GPT-2, and T5, along with tools for fine-tuning these models on domain-specific tasks. By democratizing access to advanced NLP models, Hugging Face empowers developers to push the boundaries of what’s possible in AI-driven automation, enabling applications that are smarter, more responsive, and adaptable to diverse user needs.
AutoGen: Unleashing the Power of LLMs
AutoGen isn’t just a tool; it’s a paradigm shift in how we approach automation and AI-driven interactions. By combining the robust capabilities of OpenAI’s LLMs with the versatility of Hugging Face’s transformers, AutoGen enables the creation of intelligent agents that can understand context, generate human-like responses, and adapt to dynamic user interactions. Whether you’re building customer service chatbots, automating data analysis workflows, or enhancing virtual assistants, AutoGen provides the tools and frameworks needed to accelerate development and deliver impactful AI solutions.
AutoGen Main Features
1. Agent Customization
AutoGen allows developers to create and customize agents tailored to specific tasks and workflows. This feature enables fine-tuning of agent behaviors and responses, ensuring they are optimized for different contexts and user interactions. Whether you need a customer support bot, a virtual assistant, or a data analysis tool, Agent Customization empowers you to tailor the agent’s capabilities to meet specific business needs.
2. Multi-Agent Conversation
Facilitating collaborative interactions between multiple agents, AutoGen supports complex tasks that require coordination and teamwork among different AI entities. This feature is particularly useful in scenarios where tasks span across multiple domains or require expertise from various specialized agents. Multi-Agent Conversation enhances efficiency by distributing workload and leveraging diverse skill sets within the AI ecosystem.
3. Flexible Conversation Patterns
AutoGen offers flexibility in designing conversation patterns, accommodating a wide range of workflows and interaction styles. Whether it’s linear interactions, decision trees, nested conversations, or dynamic flow control, developers can design complex workflows that mirror real-world scenarios. Flexible Conversation Patterns ensure that the AI agents can handle diverse user inputs and navigate through complex decision-making processes seamlessly.
4. Working Systems Collection
To illustrate its versatility and application across different domains, AutoGen provides a collection of pre-built working systems. These examples showcase how AutoGen can be applied to solve real-world problems in areas such as customer service, financial analysis, healthcare diagnostics, and more. The Working Systems Collection serves as a valuable resource for developers seeking inspiration or practical implementation ideas, demonstrating AutoGen’s adaptability and effectiveness in diverse use cases.
Setup
To begin implementing AutoGen, follow these setup steps:
python
# Install required libraries
!pip install openai transformers
# Import necessary libraries
import os
import openai
from transformers import pipeline
# Set up OpenAI API key
openai.api_key = os.getenv(“OPENAI_API_KEY”)
# Set up local LLM (e.g., GPT-2) from Hugging Face
local_llm = pipeline(‘text-generation’, model=’gpt2′)
Explanation:
-
Installing Libraries: Installs the openai and transformers libraries using pip for interaction with OpenAI and Hugging Face’s models.
-
Importing Libraries: Imports essential modules (os for environment variables, openai for API interaction, pipeline for model usage).
-
Setting up API Key: Retrieves and sets the OpenAI API key from environment variables for authentication.
-
Setting up Local LLM: Initializes a local language model using Hugging Face’s pipeline function, in this case, GPT-2 for text generation.
Define Agents
Define agents for simulating conversations:
python
# Define function to create agents
def create_agent(name):
return {
‘name’: name,
‘messages’: []
}
# Create Userproxy and Assistant agents
userproxy_agent = create_agent(‘Userproxy’)
assistant_agent = create_agent(‘Assistant’)
Explanation:
-
Creating Agents: Defines a function create_agent to create agent dictionaries with a name and message history.
-
Initializing Agents: Creates Userproxy and Assistant agents using the create_agent function.
Simulate Group Chat
Create functions for simulating group chat and handling external function calls:
python
# Function to simulate group chat conversation
def group_chat(user_message, assistant_message):
userproxy_agent[‘messages’].append({‘role’: ‘user’, ‘content’: user_message})
assistant_agent[‘messages’].append({‘role’: ‘assistant’, ‘content’: assistant_message})
# Function to handle external function calls
def external_function_call(function_name, *args, **kwargs):
return f”Function {function_name} called with args: {args} and kwargs: {kwargs}”
Explanation:
-
Simulating Group Chat: group_chat function appends user and assistant messages to their respective agent’s message history.
-
Handling External Function Calls: external_function_call function simulates calling an external function with given arguments.
Retrieve Agents with RAG
Implement function using Retrieval-Augmented Generation (RAG):
python
# Function to retrieve agents with RAG
def retrieve_agents_with_rag(task, code):
qa_response = local_llm(f”Answer the question: {task}”)
code_response = local_llm(f”Generate code: {code}”)
return qa_response, code_response
Explanation:
-
Retrieving Agents with RAG: retrieve_agents_with_rag uses the local LLM to generate responses for a task and code generation based on provided inputs.
Example Usage
Demonstrate the implemented functions with example interactions:
python
# Example usage
group_chat(“What is the weather today?”, “The weather today is sunny with a high of 25°C.”)
print(f”Userproxy Agent: {userproxy_agent}”)
print(f”Assistant Agent: {assistant_agent}”)
function_response = external_function_call(“weather_check”, location=”San Francisco”)
print(f”External Function Call Response: {function_response}”)
qa_task = “What is the capital of France?”
code_task = “Write a Python function to calculate factorial.”
qa_response, code_response = retrieve_agents_with_rag(qa_task, code_task)
print(f”QA Response: {qa_response}”)
print(f”Code Response: {code_response}”)
Example Usage Explanation
Simulating Group Chat Interaction:
python
group_chat(“What is the weather today?”, “The weather today is sunny with a high of 25°C.”)
-
This function simulates a conversation between a user and an assistant. The group_chat function appends the user’s message (“What is the weather today?”) to the userproxy_agent‘s message history and the assistant’s response (“The weather today is sunny with a high of 25°C.”) to the assistant_agent‘s message history. This demonstrates how AutoGen can manage simple user queries and provide informative responses.
External Function Call:
python
function_response = external_function_call(“weather_check”, location=”San Francisco”)
-
The external_function_call function simulates calling an external function named “weather_check” with a location argument set to “San Francisco”. In a real-world application, this function might fetch weather data for the specified location and return a response, showcasing AutoGen’s ability to integrate external functionalities seamlessly into its AI-driven workflows.
Retrieving Agents with RAG (Retrieval-Augmented Generation):
python
qa_task = “What is the capital of France?”
code_task = “Write a Python function to calculate factorial.”
qa_response, code_response = retrieve_agents_with_rag(qa_task, code_task)
-
The retrieve_agents_with_rag function utilizes Retrieval-Augmented Generation (RAG) to generate responses for two tasks: answering a factual question (“What is the capital of France?”) and generating code (“Write a Python function to calculate factorial.”). This demonstrates AutoGen’s capability to leverage pre-trained models and external knowledge sources to provide accurate and contextually relevant responses.
Conclusion
In summary, this guide has provided a comprehensive look into AutoGen, a powerful tool harnessing the capabilities of Large Language Models (LLMs) to automate tasks and enhance conversational interactions. By integrating OpenAI’s advanced AI models and leveraging Hugging Face’s transformers library, AutoGen empowers developers to create customized agents tailored to specific workflows. This customization capability ensures that AI agents can adapt seamlessly to diverse tasks, whether it’s customer support, data analysis, or complex decision-making processes.
Furthermore, AutoGen facilitates multi-agent conversations, enabling collaborative interactions among different AI entities. This feature enhances the tool’s utility in scenarios requiring teamwork and expertise from multiple domains. The flexibility in conversation patterns allows developers to design dynamic workflows that mirror real-world interactions, thereby optimizing user engagement and operational efficiency.
Lastly, the Working Systems Collection within AutoGen exemplifies its versatility across various industries. These pre-built systems serve as practical examples, showcasing how AutoGen can be deployed in sectors like finance, healthcare, and customer service. By following the steps outlined in this guide, developers can harness AutoGen’s capabilities to streamline operations, automate repetitive tasks, and elevate the quality of user interactions through advanced AI-driven solutions.